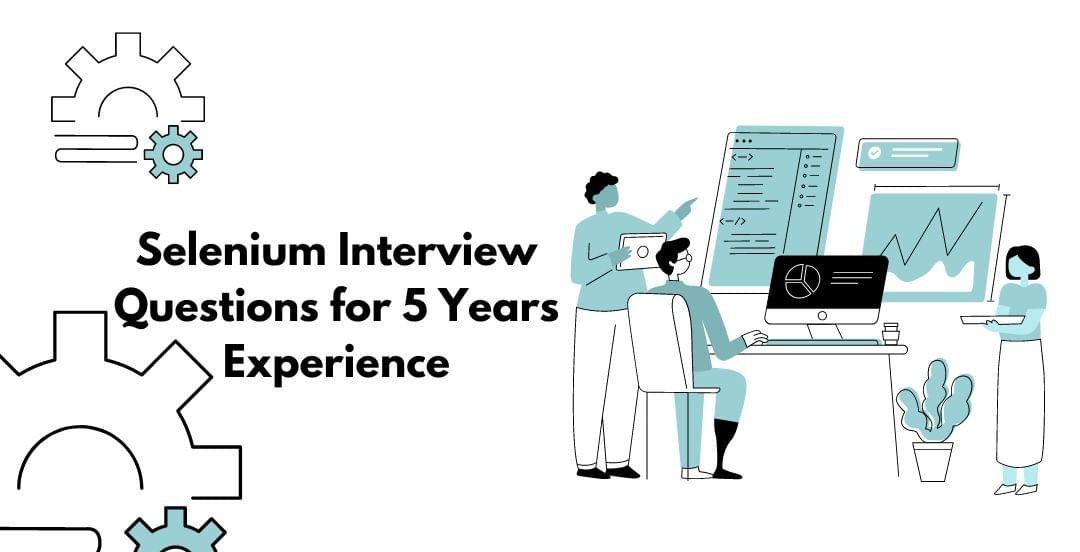
As you transition to a mid-level role in software testing, particularly with five years of experience, your expertise in Selenium should be well-developed. At this stage, interviewers will seek to assess your advanced knowledge of Selenium, your problem-solving abilities, and how you apply Selenium in practical scenarios. Consider leveraging insights from an SDET course to further enhance your preparation. Here’s a guide to some advanced Selenium interview questions you might face, along with tips on how to prepare effectively.
1. What are the different types of locators in Selenium, and when should you use each?
Selenium offers several locators for identifying web elements:
- ID: The most reliable locator due to its uniqueness. Example: `driver.findElement(By.id(“username”))`.
- Name: Useful when IDs are not available. Example: `driver.findElement(By.name(“password”))`.
- Class Name: Ideal for elements with unique class names. Example: `driver.findElement(By.className(“submit-btn”))`.
- Tag Name: Helpful for finding elements by their tag. Example: `driver.findElement(By.tagName(“button”))`.
- Link Text: Effective for locating links by their visible text. Example: `driver.findElement(By.linkText(“Forgot Password”))`.
- Partial Link Text: Similar to Link Text but allows partial matches. Example: `driver.findElement(By.partialLinkText(“Forgot”))`.
- CSS Selector: Provides flexibility by using CSS attributes. Example: `driver.findElement(By.cssSelector(“.btn.primary”))`.
- XPath: Allows navigation through XML document structures. Example: `driver.findElement(By.xpath(“//input[@type=’text’]”))`.
Preparation Tip: Be prepared to explain scenarios where each locator is ideal, considering factors such as performance, uniqueness, and maintainability.
2. How do you manage dynamic elements in Selenium?
Dynamic elements, which frequently change their attributes, can be tricky. Here’s how you can manage them:
- Use Stable Locators: Leverage attributes that are less likely to change, such as stable class names or relative XPaths.
- Dynamic XPath: Create XPaths that adapt to changing attributes. Example: `//div[contains(@id,’dynamicPart’)]`.
- Explicit Waits: Implement WebDriverWait to pause until elements are present or visible. Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
Preparation Tip: Be ready to describe your approach to handling dynamic elements and provide examples from your experience.
3. Explain the Page Object Model (POM) and its advantages.
The Page Object Model is a design pattern where a separate class represents each web page. This model offers several benefits:
- Maintainability: UI changes are confined to specific classes, minimizing impact on test scripts.
- Reusability: Actions and elements specific to a page are encapsulated within a class, making them reusable across different tests.
- Readability: Tests are more readable and easier to manage, as they utilize descriptive methods for interacting with the UI.
Preparation Tip: Be prepared to share examples of how you have used POM in your projects, and discuss its benefits and potential challenges.
4. How do you handle synchronization issues in Selenium tests?
Synchronization issues occur when tests execute faster than the application responds. Here’s how to address them:
- Implicit Waits: Establish a default wait time for all elements to be located. Example: `driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10))`.
- Explicit Waits: Use WebDriverWait to wait for specific conditions, such as element clickability.
- Fluent Waits: Allows customized polling intervals and exception handling.
Example:
Wait wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
Preparation Tip: Understand the differences between implicit, explicit, and fluent waits, and provide examples of when you have used each type effectively.
5. How do you perform cross-browser testing with Selenium?
Selenium supports cross-browser testing by allowing you to run tests across different browsers. Here’s how you can manage it:
- WebDriver Implementations: Use different WebDriver implementations for each browser (e.g., ChromeDriver, FirefoxDriver).
- Browser Capabilities: Configure capabilities specific to each browser to ensure consistent behavior.
- Parallel Execution: Utilize tools like Selenium Grid or cloud-based services such as BrowserStack or Sauce Labs to run tests concurrently across multiple browsers.
Preparation Tip: Share your experiences with cross-browser testing, including any challenges you encountered and how you addressed them.
6. Can you explain how Selenium interacts with web elements at a lower level?
Selenium interacts with web elements through the WebDriver API, which communicates with the browser’s native automation features. This involves:
- Commands and Responses: WebDriver sends commands (e.g., click, sendKeys) to the browser and receives responses.
- Browser-Specific Drivers: Each browser has a driver (e.g., ChromeDriver for Chrome) that converts WebDriver commands into browser-specific actions.
Preparation Tip: Understanding the underlying architecture of WebDriver can help you troubleshoot complex issues and enhance your test automation.
Conclusion
As you prepare for a Selenium interview with five years of experience, focus on demonstrating your advanced knowledge and practical skills. Emphasize your problem-solving capabilities, understanding of design patterns like POM, and ability to handle real-world challenges. Consider incorporating insights from SDET training to enhance your approach. Thorough preparation will showcase your expertise and help you advance in your career.
For more information: Advanced Selenium Interview Questions — 2024